Simple Network Python Script
- Mc Cube
- Jun 19, 2024
- 7 min read
Hey there Networkers! There is plenty out there to help you learn python, but there is often a struggle to see how this is "relevant" to networking, which from experience can make starting to learn difficult. What use is knowing how to calculate every prime number to a networker?
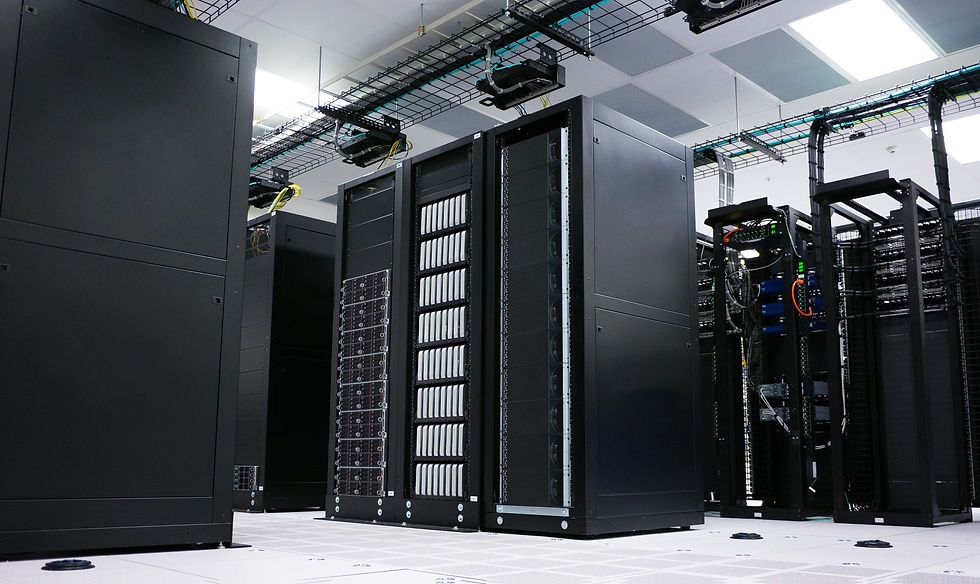
So here is a simple script that is designed to provide a little bit of relevance while providing some basic scripting elements.
Overview
I will be using VSCode to create a python script called IPCounter.py
However if you do not have an environment set up to run python code, you can always use Replit to do everything. https://replit.com/
The purpose of the script is quite simple.
Print every private RFC1918 IP address to the console
Simple Print of a single address
Let's start simple.
We will start by creating "variables". This is simply a value that we can control and "call" when we need it. In our case we will create 4 variables called "octet1", "octet2", "octet3", and "octet4". For this part of the script it will be a simple static value. we are going to print "192.168.0.0" to the console.
#input
### Setting static Values ###
octet1 = 192
octet2 = 168
octet3 = 0
octet4 = 0
### Printing out the value with a period between them ###
print (octet1, "." , octet2, "." , octet3 , "." , octet4 )
#output
192 . 168 . 0 . 0
One of the first things you may notice is that the output includes some unnecessary spacing. This is how we have used the print function. We can neaten this up using "f" strings. Which we will using in this next example.
Print all addresses in a /24 (e.g.192.168.0.0/24)
Ok, let's expand on this. We are going to tweak the value of "octet4". We basically need the above script to run 256 times, each time changing the value of octet4. Now much like static IPs being a pain, we don't want to have to do this manually! To achieve this we will create a "loop". There are different types of loops. This first we will use is a "While" loop. The logic here will be simple.
while octet4 is less than 256 print IP add 1 to octet4
Once 'octet4' reaches the value of 256 the loop will end. In this example we are also using the f strings mentioned earlier.
#input
### Setting static Values ###
octet1 = 192
octet2 = 168
octet3 = 0
octet4 = 0
### Create a while loop that prints IP and adds 1 to octet4 ###
while octet4 < 256:
print (f"{octet1}.{octet2}.{octet3}.{octet4}")
octet4 += 1
When you run this script the output should start firing away starting at 192.168.0.0 ending at 192.168.0.255
Print all private Class C addresses
You DevNet Guru! You are already punting out all addresses in a single /24 in less than a second! you must be seeing the advantage of this in networking already!
Here we need to introduce a really interesting concept a loop within a loop! We are going Infinity War Avengers on this badboy! We need to take a minute to appreciate the logic here. We need the code to act similar to a clock. Once we cycle through all values in octet4, we need the process to repeat but to have octet3 increment by only 1.
This exercise will demonstrate the importance of indentation and the order of operation.
with Octet3 being the "minutes hand" of our digital IP clock it is hierarchically higher than 'Octet4' (the seconds hand). So our program needs to work with this logic. The While loop worked well last time, but will start to get messy now. Instead we will use a "FOR" loop. Instead of having octet 3 be statically defined as "octect3 = 0" we will use the For loop with a range operator.
The logic will be as follows.
For octet3 use all values between 0 and 256 While octet4 is less than 256 print IP address add 1 to octet4
Notice we no don't need to add 1 to octet3 each time? This is because the range operator is kinda doing it for us. We start at 0, while at 0, octet 4 runs through its process. When octet 4 comes to 255, Octet3 moves in to the next value within the range, and it starts again!
#Input
### Setting static Values ###
octet1 = 192
octet2 = 168
### Creating the Range loop for octet3 ###
for octet3 in range (256):
octet4 = 0
### Create a while loop that prints IP and adds 1 to octet4 ###
while octet4 < 256:
print (f"{octet1}.{octet2}.{octet3}.{octet4}")
octet4 += 1
If you run this well done you absolute legend! You have just successfully printed over 65 thousand IPs in probably under a second. You are on the verge of being an automation master!
Turn it into a function
We are going to need to neaten up our code just a little, what a great opportunity to introduce functions!
Turning a piece of code in to a function can be tricky, but this one will be easy as we don't need to pass any information in to the function, just let it run (as we have with the script so far).
It is really easy.
Above our code we will enter the function keyword "def" followed by the name we want to give our function. Let's call it "ClassC" Every line of the existing code will need to be indented (by using the Tab key).
Finally we also need to call the new function at the bottom of the script, if we didn't the script will run but this function will never been called, meaning it wont actually do anything.
It should look like this when done.
#Input
### Creating ClassC function ###
def ClassC():
### Setting static Values ###
octet1 = 192
octet2 = 168
### Creating the Range loop for octet3 ###
for octet3 in range (256):
octet4 = 0
### Create a while loop that prints IP and adds 1 to octet4 ###
while octet4 < 256:
print (f"{octet1}.{octet2}.{octet3}.{octet4}")
octet4 += 1
### Call function to run in script ###
ClassC()
Well done your first function, right now I bet you are feeling like Tony Stark when he was building the Iron Man suit in his garage! It's early days but this $h!7 works!
Create a ClassB function
Class B is by far my favourite, mainly because it reminds me of me at school, awkward and always trying to throw people off with weird comments. WELL NO MORE for you, you DevNet Avenger!
We are going to recycle the above code into another function. So highlight every part of the ClassC function including the "def" line, and paste it directly underneath. Now we just need to change a few details.
We do need to add an extra layer of logic. Another for loop, that this time wont loop through all values between 0 and 256, but instead between values 16 to 32. The logic doesn't change much, we are just adding an extra layer above.
For octet2 use all values between 16 and 32 For octet3 use all values between 0 and 256 While octet4 is less than 256 print IP address add 1 to octet4
Some of the details we need to change.
We should change the name of our function to ClassB
Octet1 now needs to = 172.
Add new loop
Indent all lines from octet3 down
We need to call the new function act the bottom of the script.
#Input
### ^^^ ClassC function above ^^^ ###
### Creating ClassB function ###
def ClassB():
### Setting static Values ###
octet1 = 172
### Creating the range loop for octet2 ###
for octet2 in range (16,32):
### Creating the Range loop for octet3 ###
for octet3 in range (256):
octet4 = 0
### While loop that prints IP and adds 1 to octet4 ###
while octet4 < 256:
print (f"{octet1}.{octet2}.{octet3}.{octet4}")
octet4 += 1
### Call function to run in script ###
ClassC()
ClassB()
Amazing! Congratulations you are effectively as good as 90% of the software developers out there who simply recycle code from stack overflow!
Call to action!
Come on Team Avengers! Your mission is to see if you can create the function, that will print out all ClassA private addresses! This blog should have comments turned on so feel free to throw your reply in the reply, along with what your DevNet Avenger Name would be!
Can it configure?
Perhaps we can make this a little more useful for you.
Let's imagine a weird scenario where you need to configure a switch. With 255 vlans, all with an Switched Virtual Interface (SVI) that belong to a /24 network.
Each SVI requires an IP address. If we use the ClassC function, we can tweak it, so that we give every SVI the .5 address from every /24 within the ClassC range! Sound Awesome! You bet your ass it does!
What will we add to this function? Well we need to add a value for the vlan, This will needed to be increased by every loop. For security we will start at vlan2 and just to keep it interesting we will increase it by 2 at the end of the script. So we should get VLAN 2,4,6,8,...,512
Then we need to set octet4 to the static value of 5, this way we get IPs:
192.168.1.5,
192.168.2.5,
192.168.3.5,
...,
192.168.255.5
Finally we want to print the commands we would need to configure this
interface vlan x ip address 192.168.y.5 255.255.255.0
The only extra thing I will draw your attention to is the "\n" within the 2 print lines. "\n" is simply pythonic for "Carriage Return" or "new line" So this just makes the output a little neater, providing a line break before each line of output. There are neater ways to do this. but I wanted it to stand out for you at the moment.
#Input
### New function to create switch IP addresses ###
def switchIP():
# Add a VLAN counter ###
vlan = 1
### Create static octet1&2 values ###
a = 192
b = 168
### Create range function for octet3 ###
for c in range(256):
### Set octet4 with static value ###
d = 5
### print commands needed ###
print ("\n")
print(f"interface vlan {vlan}")
print (f" ip address {a}.{b}.{c}.{d} 255.255.255.0")
vlan += 2
switchIP()
And what will your output look like?
Like THIS!!!
#Output
... [output omitted] ...
interface vlan 508
ip address 192.168.253.5 255.255.255.0
interface vlan 510
ip address 192.168.254.5 255.255.255.0
interface vlan 512
ip address 192.168.255.5 255.255.255.0
That's right! Usable, copy and pastable code!
I seriously hope you enjoyed this introduction to coding in python. Please let me know how you get on printing all class A addresses! Any questions feel free to ask.
Comments